Using WordPress as a Headless CMS with React
Co-authored by Kostas Minaidis and ChatGPT | Last updated: 26.12.2024
Introduction
Modern web applications thrive on dynamic, flexible, and efficient communication between systems. Today, we’ll explore how REST APIs, JSON data, Headless CMS platforms, and React SPAs (Single Page Applications) fit together. Let’s dive into these essential concepts and build something practical along the way.
REST APIs and JSON Data: The Backbone of Modern Web Apps
REST (Representational State Transfer) APIs are a way for web applications to communicate. They allow apps to request and send data using HTTP methods like GET, POST, and DELETE. Most APIs return data in JSON (JavaScript Object Notation) format, which is lightweight and easy to use in modern programming languages.
Example:
Requesting a list of users from an API might look like this:
fetch('https://api.example.com/users')
.then(response => response.json())
.then(data => console.log(data));
The response might look like this:
[
{ "id": 1, "name": "John Doe" },
{ "id": 2, "name": "Jane Smith" }
]
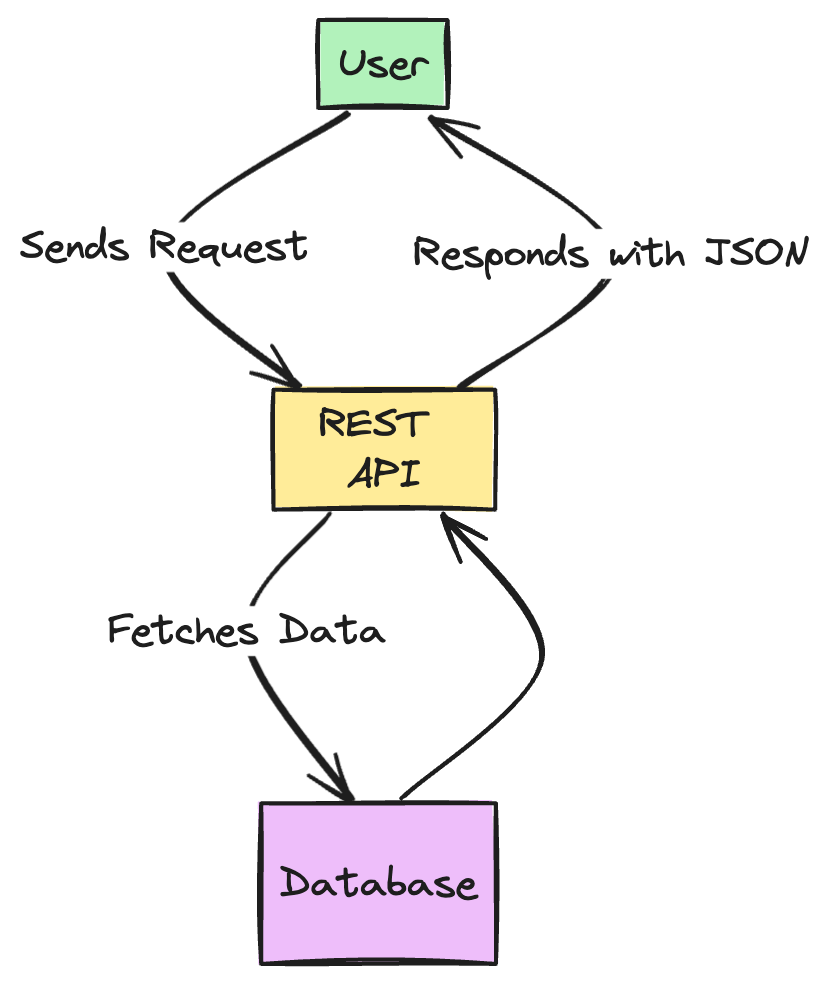
(Image: REST APIs and JSON. Click to Zoom)
The WordPress REST API
The WordPress REST API brings RESTful principles to WordPress, making its data accessible to external applications. This API lets developers retrieve posts, pages, categories, and more in JSON format.
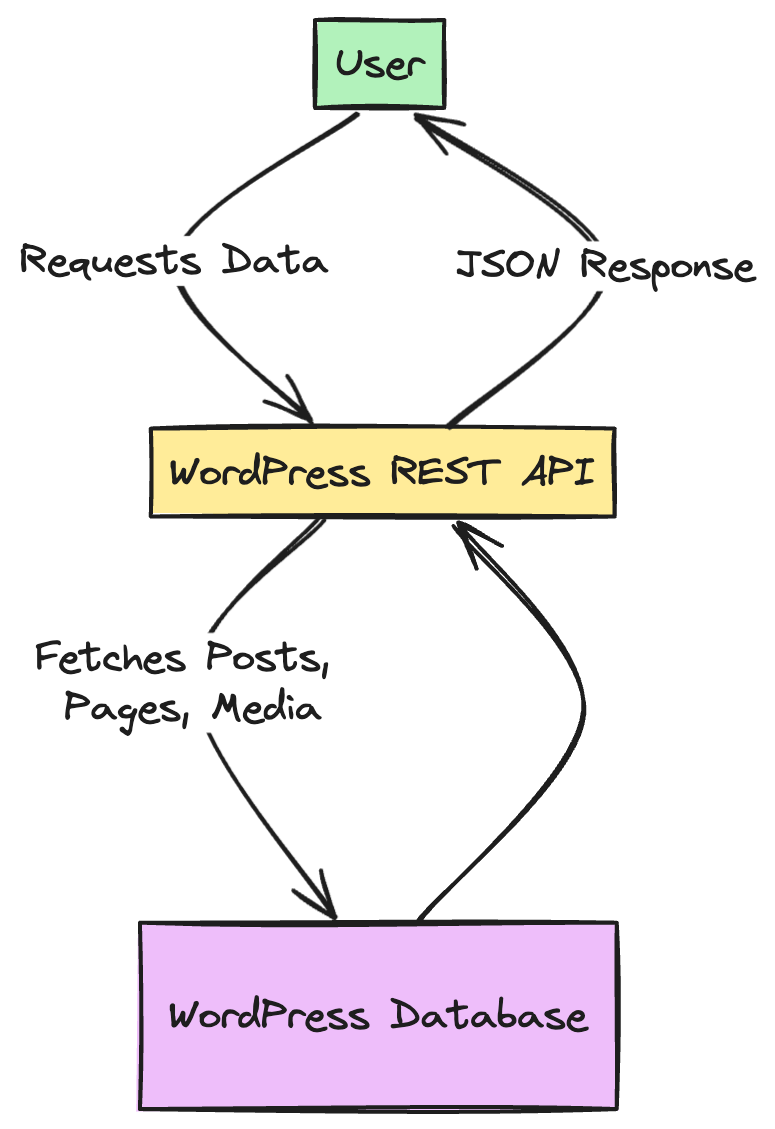
(Image: The WordPress REST API. Click to Zoom)
By default, the WordPress REST API is enabled on all WordPress installations (starting with version 4.7). You don’t need to take extra steps to turn it on unless you’ve explicitly disabled it.
The base endpoint for the API is: https://your-site-url/wp-json/
Testing the API:
Visit https://your-site-url/wp-json/wp/v2/posts in your browser or use tools like Postman or curl to verify that the API is working.
The API endpoints follow a logical structure based on your WordPress content types. Examples include:
- Posts: /wp-json/wp/v2/posts
- Pages: /wp-json/wp/v2/pages
- Categories: /wp-json/wp/v2/categories
- Users: /wp-json/wp/v2/users
Example:
Fetching blog posts from a WordPress site:
fetch('https://example.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(posts => console.log(posts));
A sample response:
[
{
"id": 101,
"title": { "rendered": "Welcome to My Blog" },
"content": { "rendered": "<p>Hello world!</p>" }
}
]
Headless CMS
A Headless CMS (Content Management System) provides content storage and management without dictating how the content is displayed. Unlike traditional CMS platforms, it only serves content through APIs, allowing developers full control over the front-end experience.
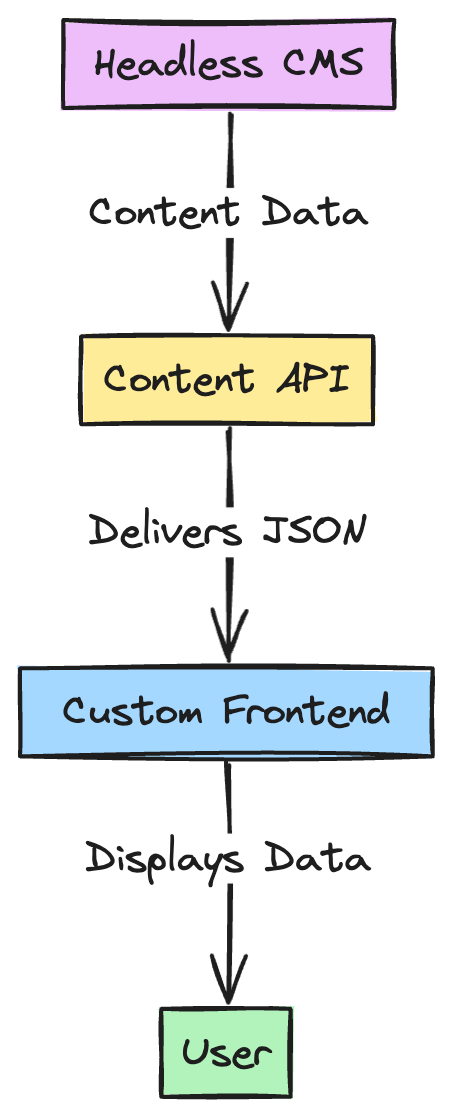
(Image: Headless CMS, an overview. Click to Zoom)
WordPress as a Headless CMS
Using the WordPress REST API, you can decouple the WordPress back-end from its front-end. This makes WordPress a Headless CMS—an ideal setup for modern applications where a JavaScript framework like React is used to build the user interface.
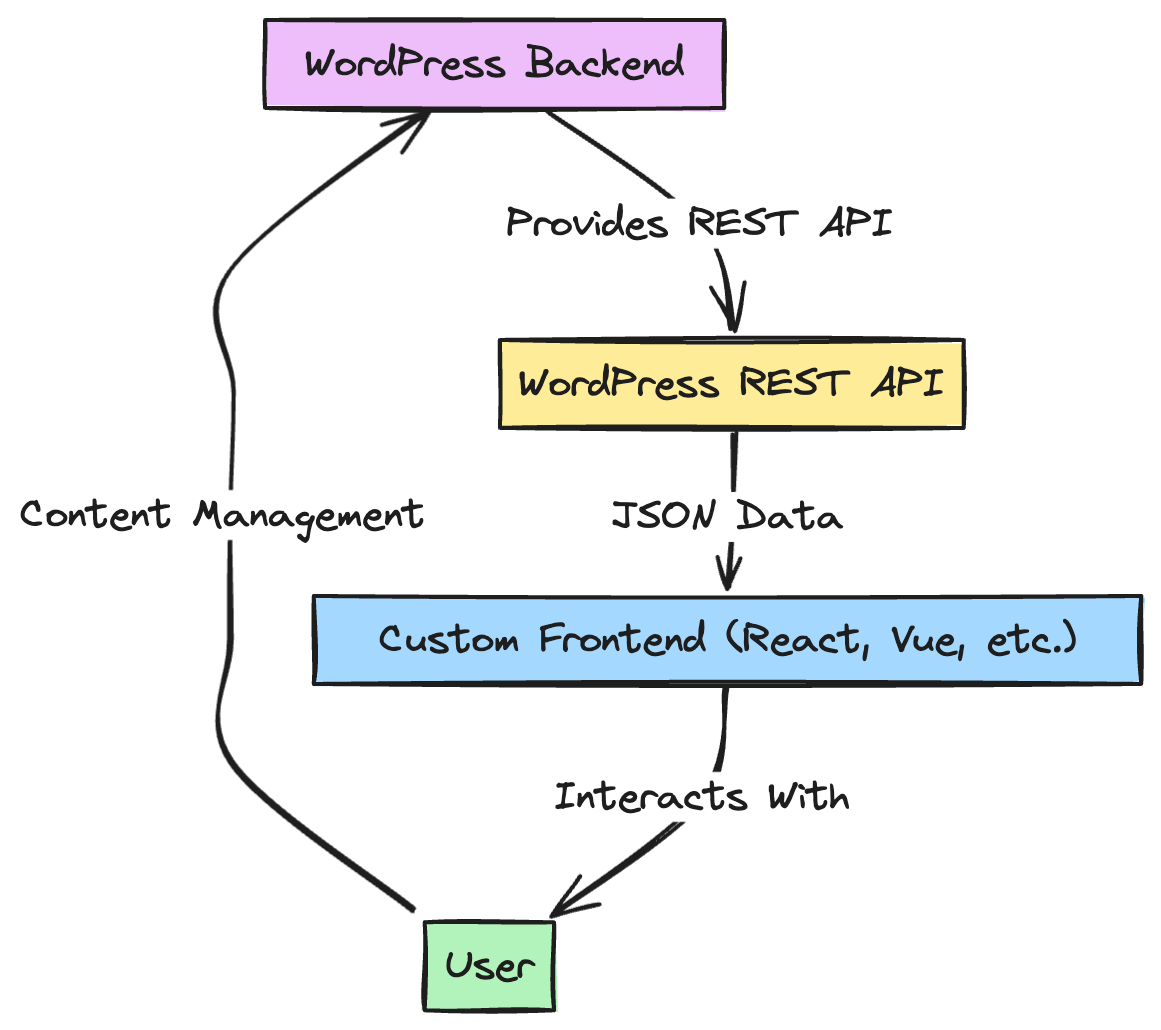
(Image: WP as Headless CMS. Click to Zoom)
Example Workflow:
- Use WordPress to create and manage content.
- Let React fetch the content via the WordPress REST API.
- Render the content using React.
Building a React SPA with WordPress Data
Now, let’s put it all together by creating a simple React Single Page Application (SPA) that fetches and displays posts from a WordPress site.
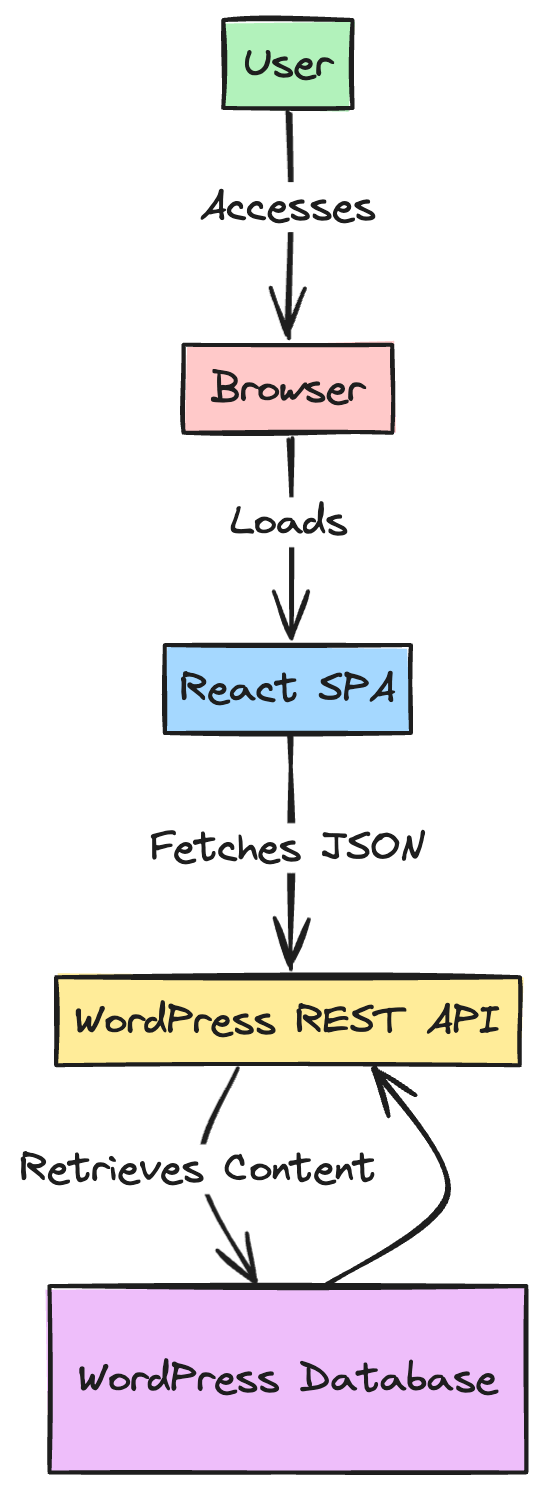
(Image: React interacting with a Headless WP. Click to Zoom)
Step 1: Initialize a React Project
Use vite to set up your React environment:
npm create vite@latest wp-react-spa -- --template react
cd wp-react-spa
npm install
npm run dev
Step 2: Fetch WordPress Posts
Update the App.js
file to fetch and display posts:
import React, { useState, useEffect } from 'react';
function App() {
const [posts, setPosts] = useState([]);
useEffect(() => {
fetch('https://example.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(data => setPosts(data));
}, []);
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map(post => (
<li key={post.id}>
<h2>{post.title.rendered}</h2>
<div dangerouslySetInnerHTML={{ __html: post.content.rendered }} />
</li>
))}
</ul>
</div>
);
}
export default App;
Step 3: Hosting the SPA
-
Build the React app:
npm run build
-
Host it on platforms like Netlify, GitHub Pages, Vercel or your preferred hosting service.
-
Ensure the WordPress REST API is publicly accessible or uses secure tokens for restricted access.
Conclusion
With REST APIs, JSON data, and the power of Headless CMS platforms like WordPress, you can build flexible, fast, and modern web applications. Combining WordPress with React opens up endless possibilities for creating dynamic, user-centric experiences.
How this article was created
- The diagrams were generated by ChatGPT using the mermaid format as instructed. They were converted to Excalidraw drawings using the Mermaid-to-Excalidraw tool, in order to be easily manipulated, styled and exported to jpg images.